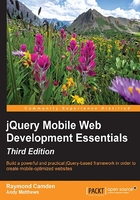
Working with multiple files
In an ideal world, we could build an entire website with one file. We would never have to perform revisions, and we would be done with every project by 2 P.M. on Fridays. But in the real world, we have to deal with lots of files, lots of revisions, and, unfortunately, lots of work. In the earlier code listing, you saw how we could include two pages within one file. jQuery Mobile handles this easily enough. But you can imagine that this would get unwieldy after a while. While we could include 10, 20, or even 30 pages, this will make the file difficult to work with and make the initial download for the user that much slower.
To work with multiple pages and files, all we need to do is make a simple link to other files in the same domain as our first file. We can even combine the first technique (two pages in one file) with links to the other files. In listing 2-2
, we've modified the first example to add a link to a new page (as mentioned previously, we are only listing the relevant portion of the page):
Listing 2-2:test2.html <p data-role="page" id="homePage"> <p data-role="header"><h1>Welcome</h1></p> <p role="main" class="ui-content"> <p> Welcome to our first mobile web site. It's going to be the best site you've ever seen. Once we get some content. And a business plan. But the hard part is done! </p> <p> Find out about our wonderful <a href="products.html">products</a>. </p> <p> You can also <a href="#aboutPage">learn more</a> about Megacorp. </p> </p> <p data-role="footer"> <h4>Copyright Megacorp © 2015</h4> </p> </p> <p data-role="page" id="aboutPage"> <p data-role="header"><h1>About Megacorp</h1></p> <p role="main" class="ui-content"> <p> This text talks about Megacorp and how interesting it is. Most likely though you want to <a href="#homePage">return</a> to the home page. </p> </p> <p data-role="footer"> <h4>Copyright Megacorp © 2015</h4> </p> </p>
Now, let's look at listing 2-3
, our Products page:
Listing 2-3: products.html <!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Products</title> <link rel="stylesheet" href="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.css" /> <script type="text/javascript" src="http://code.jquery.com/jquery-2.1.3.min.js"></script> <script type="text/javascript" src="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.js"></script> </head> <body> <p data-role="page" id="productsPage"> <p data-role="header"><h1>Products</h1></p> <p role="main" class="ui-content"> <p> Our products include: </p> <ul> <li>Alpha Series</li> <li>Beta Series</li> <li>Gamma Series</li> </ul> </p> </p> </body> </html>
Our Products page is rather simple, but notice that we included the jQuery and jQuery Mobile resources on top. Why? I mentioned earlier that jQuery Mobile is going to use AJAX to load your additional pages. If you open test2.html
in any modern browser, you can see this for yourself using developer tools. Clicking on the link for Products will fire an XML HTTP Request (XHR), but generally, it just means an AJAX call, as shown in the following screenshot from Chrome's DevTools:

That's neat. But what happens when someone bookmarks the application? Let's now take a look at how jQuery Mobile handles URLs and navigation.
Tip
What are browser developer tools?
All modern browsers have built-in tools to help you build web pages. These tools allow you to inspect and manipulate the DOM, pause and debug JavaScript execution, and view network activity and errors.