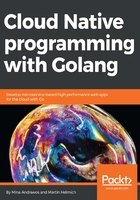
RESTful Web APIs
REST stands for Representational State Transfer. REST is simply a way for different services to communicate and exchange data. The core of the REST architecture consists of a client and a server. The server listens for incoming messages, then replies to it, whereas the client starts the connection, then sends messages to the server.
In the modern web programming world, RESTful web applications use the HTTP protocol for communication. The RESTful client would be an HTTP client, and the RESTful server would be the HTTP server. The HTTP protocol is the key application layer communication protocol that powers the internet, which is why RESTful applications can also be called web applications. The communication layer of the RESTful applications is often simply referred as RESTful APIs.
REST APIs allow applications developed in various types of platforms to communicate. This includes other microservices in your application that run on other operating systems, as well as client applications running on other devices. For example, a smartphone can communicate with your web services reliably via REST.

Web RESTful API
To understand how RESTful applications work, we will first need to gain a decent understanding of how the HTTP protocol works. HTTP is an application-level protocol used for data communications all over the web, the clouds, and the world of modern microservices.
HTTP is a client-server, request-response protocol. This means that the data flow works as follows:
- An HTTP client sends a request to an HTTP server
- The HTTP server listens to incoming requests, then responds to them as they come

Requests and response
An HTTP client request is typically one of two things:
- The client is requesting a resource from the server
- The client is requesting to add/edit a resource on the server
The nature of the resource depends on your application. For example, if your client is a web browser trying to access a web page, then your client will send a request to the server asking for an HTML web page. The HTML page would be the resource returned within the response of the HTTP web server to the client.
In the world of communicating microservices, REST applications usually use the HTTP protocol in combination with the JSON data format in order to exchange data messages.
Consider the following scenario: In our MyEvents application, one of our microservices needs to obtain the information of an event (duration, start date, end date, and location) from another microservice. The microservice in need of the information will be our client, whereas the microservice providing the information will be our server. Let's assume that our client microservice has the event ID, but needs the server microservice to provide the information of the event that belongs to that ID.
The client will send a request inquiring about the event information via the event ID; the server will respond with the information enclosed in the JSON format, as follows:

JSON document with response
This description sounds simple; however, it doesn't deliver the full picture. The inquiring part of the client needs more elaboration in order for us to understand how REST APIs really work.
There are two primary pieces of information that a REST API client request needs to specify in order to declare its intent—the request URL and the request method.
The request URL is the address of the resource at the server that the client seeks. An URL is a web address, an example of a REST API URL would be http://quotes.rest/qod.json, which is an API service that returns a quote for the day.
In our scenario, the MyEvents client microservice can send an HTTP request to the http://10.12.13.14:5500/events/id/1345 URL to inquire about event ID 1345.
The request method is basically the type of operation that we would like to execute. That could range from a request to obtain a resource to a request to edit a resource, add a resource, or even delete a resource. In the HTTP protocol, there are multiple types of methods that need to be part of the client request; the following are some of the most common methods:
- GET: A very common HTTP method in web applications; this is how we request a resource from our HTTP web servers; this is the request type we would use in our scenario to request the data of event ID 1345.
- POST: The HTTP method we would use to update or create a resource.
Let's assume that we would like to update a piece of information that belongs to event ID 1345 using POST, then we'd send a POST request to relative URL ../events/id/1345 with new event information in the request body.
If on the other hand, we would like to create a new event that has an ID of 1346, we shouldn't send a POST request to ../events/id/1346 with the new event information because the ID doesn't yet exist. What we should do is just send a POST request to .../events and attach all the new event information in the request body.
- PUT: The HTTP method to create or overwrite a resource.
Unlike POST, the PUT request can be used to create a new resource by sending a request to a resource ID that didn't exist from before. So, for example, if we want to create a new event with an ID 1346, we can send a PUT request to ../events/id/1346, and the web server should create the resource for us.
PUT can also be used to fully overwrite an existing resource. So, unlike POST, we shouldn't use PUT to just update a single piece of information of a resource.
- DELETE: It is used to delete a resource. For example, if we send a delete request to the relative URL ../events/id/1345 of the web server, the web server will then delete the resource from the database.