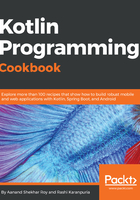
上QQ阅读APP看书,第一时间看更新
How to do it...
First, let's create a file, name it whenWithRanges.kt, and follow these steps:
- Let's try a basic when statement to understand how it works:
fun main(args: Array<String>) {
val x = 12
when(x){
12 -> println("x is equal to 12")
4 -> println("x is equal to 4")
else -> println ("no conditions match!")
}
}
So basically, this code block works like a switch case statement, and it can also be implemented using an if statement.
- Now, let's see if x lies between 1 to 10 or outside it:
fun main(args: Array<String>) {
val x = 12
when(x){
in (1..10) -> println("x lies between 1 to 10")
!in (1..10) -> println("x does not lie between 1 to 10")
}
}
- Let's see what else we can do. In the following example, we will work with different types of conditions that can be used inside a when statement:
fun main(args: Array<String>) {
val x = 10
when(x){
magicNum(x) -> println("x is a magic number")
in (1..10) -> {
println("lies between 1 to 10, value: ${if(x < 20) x else 0}")
}
20,21 -> println("$x is special and has direct exit access")
else -> println("$x needs to be executed")
}
}
fun magicNum(a: Int): Int {
return if(a in (15..25)) a else 0
}
- Finally, let's try a more complicated example of using data classes. In this example, we will see how to use when with objects:
fun main(args: Array<String>) {
val x = ob(2, true, 500)
when(x.value){
magicNum(x.value) -> println("$x is a magic number and ${if(x.valid) "valid" else "invalid"}")
in (1..10) -> {
println("lies between 1 to 10, value: ${if(x.value < x.max) x.value else x.max}")
}
20,21 -> println("$x is special and has direct exit access")
else -> println("$x needs to be executed")
}
}
data class ob(val value: Int, val valid: Boolean, val max: Int)
fun magicNum(a: Int): Int {
return if(a in (15..25)) a else 0
}
Here's how it looks after compiling and running the program:

Try playing around with the values and logic to see what else you can do with such a small block of code in Kotlin using when.