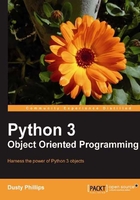
Making it do something
Now, having objects with attributes is great, but object-oriented programming is really about the interaction between objects. We're interested in invoking actions that cause things to happen to those attributes. It is time to add behaviors to our classes.
Let's model a couple of actions on our Point
class. We can start with a method called reset
that moves the point to the origin (the origin is the point where x
and y
are both zero). This is a good introductory action because it doesn't require any parameters:
class Point: def reset(self): self.x = 0 self.y = 0 p = Point() p.reset() print(p.x, p.y)
That print statement shows us the two zeros on the attributes:
0 0
A method in Python is identical to defining a function. It starts with the keyword def
followed by a space and the name of the method. This is followed by a set of parentheses containing the parameter list (we'll discuss the self
parameter in just a moment), and terminated with a colon. The next line is indented to contain the statements inside the method. These statements can be arbitrary Python code operating on the object itself and any parameters passed in as the method sees fit.
The one difference between methods and normal functions is that all methods have one required argument. This argument is conventionally named self
; I've never seen a programmer use any other name for this variable (convention is a very powerful thing). There's nothing stopping you, however, from calling it this
or even Martha
.
The self
argument to a method is simply a reference to the object that the method is being invoked on. We can access attributes and methods of that object as if it were any other object. This is exactly what we do inside the reset
method when we set the x
and y
attributes of the self
object.
Notice that when we call the p.reset()
method, we do not have to pass the self
argument into it. Python automatically takes care of this for us. It knows we're calling a method on the p
object, so it automatically passes that object to the method.
However, the method really is just a function that happens to be on a class. Instead of calling the method on the object, we could invoke the function on the class, explicitly passing our object as the self
argument:
p = Point()
Point.reset(p)
print(p.x, p.y)
The output is the same as the previous example because, internally, the exact same process has occurred.
What happens if we forget to include the self argument in our class definition? Python will bail with an error message:
>>> class Point: ... def reset(): ... pass ... >>> p = Point() >>> p.reset() Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: reset() takes no arguments (1 given)
The error message is not as clear as it could be ("You silly fool, you forgot the self
argument" would be more informative). Just remember that when you see an error message that indicates missing arguments, the first thing to check is whether you forgot self
in the method definition.
So how do we pass multiple arguments to a method? Let's add a new method that allows us to move a point to an arbitrary position, not just the origin. We can also include one that accepts another Point
object as input and returns the distance between them:
import math class Point: def move(self, x, y): self.x = x self.y = y def reset(self): self.move(0, 0) def calculate_distance(self, other_point): return math.sqrt( (self.x - other_point.x)**2 + (self.y - other_point.y)**2) # how to use it: point1 = Point() point2 = Point() point1.reset() point2.move(5,0) print(point2.calculate_distance(point1)) assert (point2.calculate_distance(point1) == point1.calculate_distance(point2)) point1.move(3,4) print(point1.calculate_distance(point2)) print(point1.calculate_distance(point1))
The print statements at the end give us the following output:
5.0 4.472135955 0.0
A lot has happened here. The class now has three methods. The move
method accepts two arguments, x
and y
, and sets the values on the self
object, much like the old reset
method from the previous example. The old reset
method now calls move
, since a reset is just a move to a specific known location.
The calculate_distance
method uses the not-too-complex Pythagorean Theorem to calculate the distance between two points. I hope you understand the math (**
means squared, and math.sqrt
calculates a square root), but it's not a requirement for our current focus: learning how to write methods.
The example code at the end shows how to call a method with arguments; simply include the arguments inside the parentheses, and use the same dot notation to access the method. I just picked some random positions to test the methods. The test code calls each method and prints the results on the console. The assert
function is a simple test tool; the program will bail if the statement after assert
is False
(or zero, empty, or None
). In this case, we use it to ensure that the distance is the same regardless of which point called the other point's calculate_distance
method.