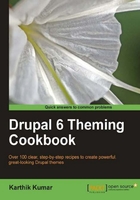
This recipe provides the PHP code that allows the rotation of themes based on the day of the week.
As we have seen in other recipes in this chapter, a number of sites use an "odds and ends" module to handle tweaks and customizations particular to the site. We will be using the mysite.module
created earlier in this chapter to hold our customizations. It is assumed that the module is available and already enabled.
Open the mysite
module file and paste the following code into it:
/** * Implementation of hook_init(). */ function mysite_init() { global $custom_theme; // An array of themes for each day of the week. // These themes do not have to be enabled. $themes = array(); $themes[0] = 'garland'; $themes[1] = 'minnelli'; $themes[2] = 'bluemarine'; $themes[3] = 'pushbutton'; $themes[4] = 'chameleon'; $themes[5] = 'marvin'; $themes[6] = 'mytheme'; // Get the current day of the week in numerical form. $day = date("w"); // Override current theme based on day of the week. $custom_theme = $themes[$day]; }
Save the file and then refresh the page on the Drupal site to see this snippet at work. Due to the nature of this feature, it might be necessary to fiddle with the computer's date settings to simulate different days of the week during testing.
The function hook_init ()
is run on every page without exception. It is also run before Drupal gets to the theming stage when it displays every page. Consequently, this is the best place to override the theme to use.
We are overriding the site's theme by manipulating a global variable named $custom_theme
. If it is set, then Drupal changes the theme being used to the value in this variable. Now that we know how to change the theme, it is just a question of when to do it. Since we are looking to change it based on the day of the week, we are going to take advantage of a feature of PHP's date()
function which returns the day of the week as a number between zero and six.
// Get the current day of the week in numerical form. $day = date("w");
We now map the $day
variable to the $themes
array which also has elements from 0 to 6 associated with seven enabled themes and, as a result, we obtain the equivalent theme for that day of the week. Finally, assigning our result to the $custom_theme
variable overrides the default theme with the theme for the current day of the week.
The PHP code used in this recipe can be easily modified to display a random theme on every page load.