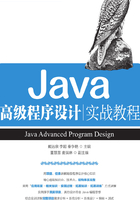
上QQ阅读APP看书,第一时间看更新
1.3 任务实施
任务 计算员工的月工资
1. 任务需求
某公司分为多个部门,每个部门有一个经理和多个员工,每个员工根据职称发基本工资。员工的工资由基本工资、日加班工资、日缺勤工资等组成。具体需求如下所示。
• 员工的基本信息,包括部门、职务、职称以及工资记录等信息。
• 能记录员工的每一个职称信息,并授予相应的职称,系统在计算员工工资的时候选取职称对应的最高职称津贴。
2. 任务分析
问题域中涉及多个类,包括职员类Staffer、经理类Manager、测试类TestEmployee。
• Staffer类:通过此类封装定义计算职员基本工资方法。
• Manager类:通过此类封装定义计算经理基本工资方法。
• TestEmployee类:调用方法并实现结果输出。
其类图关系如图1-1所示。

图1-1 类图关系
3. 任务实现
package com.daiinfo.seniorjava.ken1.implment; / ** *封装员工的信息和操作 * @author daiyuanquan * * / public class Employee { String ID; String name; int workdays;//工作天数 int overtimedays;//加班天数 int absencedays;//缺勤天数 double salary;//月工资 / ** * 构造函数 * / public Employee(String ID) { // TODO Auto-generated constructor stub this.ID=ID; } / ** * 构造函数 * / public Employee(String ID,String name) { // TODO Auto-generated constructor stub this.ID=ID; this.name=name; } / ** * 计算员工的工资 * @param workdays 工作天数 * @param overtimedays 加班天数 * @param absencedays 缺勤天数 * @return 返回月工资总数 * / double calculateCount(int workdays,int overtimedays,int absencedays){ double count=0.0; count=80.0*workdays+80*overtimedays-30*absencedays; return count; } / ** * 转换字符串 * / public String toString(){ return name+"\t"+salary; } }
package com.daiinfo.seniorjava.ken1.implment; public class Staff extends Employee{ double bassewages=2000; double dailywages=50;//日工资 String department; String technicaltitle; / ** * 构造函数 * / public Staff(String ID,String name,String department,String technicaltitle) super(ID,name); this.department=department; this.technicaltitle=technicaltitle; } / ** * 计算员工的工资 * @param workdays 工作天数 * @param overtimedays 加班天数 * @param absencedays 缺勤天数 * @return 返回月工资总数 * / double calculateCount(int workdays,int overtimedays,int absencedays){ double count=0.0; count=bassewages+dailywages*overtimedays-dailywages*absencedays; return count; } / ** * 转换字符串输出信息 * / public String toString(){ return name+"\t"+salary; } }
package com.daiinfo.seniorjava.ken1.implment; public class Manager extends Employee { double basewages=3000; String department;//所在部门 String positions; / ** * 构造函数 * @param ID * @param name * @param department * / public Manager(String ID,String name,String department,String positions) { // TODO Auto-generated constructor stub super(ID, name); this.department=department; this.positions=positions; } / ** * 计算经理的工资 * @param workdays 工作天数 * @param overtimedays 加班天数 * @param absencedays 缺勤天数 * @return 返回月工资总数 * / double calculateCount(int workdays,int overtimedays,int absencedays){ double count=0.0; count=basewages+20*overtimedays-30*absencedays; return count; } }
package com.daiinfo.seniorjava.ken1.implment; / ** * 测试类的引用 *@Copyright: Copyright (c) 2017 *@Company: daiinfo * *@author daiyuanquan *@version 1.0 *@since 1.0 * / public class TestEmployee { public static void main(String[] args) { // TODO Auto-generated method stub Manager manager=new Manager("001", "张三", "开发部","经理"); double countsalary=manager.calculateCount(22, 3, 0); System.out.println(countsalary); Staff staff=new Staff("2001", "王好", "财务部", "会计师"); double salary=staff.calculateCount(20, 5, 1); System.out.println(salary); } }
运行结果如图1-2所示。

图1-2 运行结果